The C program to process marks of student and display grade checks for multiple conditions on the input marks and then decides the grade of a student.
This program is written using Dev C++ compiler version 4.9.9.2 installed on Windows 7 64-bit computer. You can use any standard C compiler and this program should work.
To help you understand the program we have the following sections – problem definition, a flowchart of the program, the source code for the program and a verified output. When you practice the program by yourself, make sure that you compare the output value from this article.
Problem Definition
This program is a simple C program that makes use of structures. The program when executed asks for “Total number of students for whom marks should be processed”. Upon receiving the number of student value, it asks for each student details such as
- Rollno
- Name
- Marks
When all the details are entered it is stored in an array of structures.
The marks of student is used to process the grades and stored in the variable grade of each student. The program prints the results for each student.
The grade is calculated based on the following logic.
If grade is less than or equal to 50, the student gets 'F',
If grade is greater than 50, but less than or equal to 55, student gets a 'D',
If grade is greater than 55, but less than or equal to 60, student gets a 'C',
If grade is greater than 60, but less than or equal to 75, student gets a 'B',
if grade is grater than 75, but less than or equal to 90, then student gets 'A',
if grade is above 90, the student get the highest grade - 'A+'.
You may change the evaluation criteria as per your need.
Program Code – Processing Student Marks and Display Results
/* Program to process marks of students and display Grades */ #include <stdio.h> #include <conio.h> struct student{ char name[25]; int rollno; int marks; char grade; }; main() { struct student stud[15]; int i,n; /* Get the number of students */ printf("ENTER THE NUMBER OF STUDENTS:"); scanf("%d",&n); /* Get the value of Students marks*/ for(i=0;i<n;i++) { printf("ENTER STUDENT INFORMATION:\n"); printf("NAME:"); scanf("%s",&stud[i].name); printf("ROLLNO:"); scanf("%d",&stud[i].rollno); printf("MARKS(In Percentage):"); scanf("%d",&stud[i].marks); printf("\n"); } /* Print student information*/ for(i=0;i<n;i++) { if(stud[i].marks <= 50) { stud[i].grade = 'F'; } else if(stud[i].marks > 50 && stud[i].marks <= 55) { stud[i].grade = 'D'; } else if(stud[i].marks > 55 && stud[i].marks <= 60) { stud[i].grade = 'C'; } else if(stud[i].marks > 60 && stud[i].marks <= 75) { stud[i].grade = 'B'; } else if (stud[i].marks > 75 && stud[i].marks <= 90) { stud[i].grade = 'A'; } else if(stud[i].marks > 90) { stud[i].grade = 'S'; } } for(i = 0;i<40;i++) printf("_");printf("\n"); printf("Name\tRollNo\tMarks\tGrade\n"); for(i = 0;i<40;i++) printf("_");printf("\n"); for(i=0;i<n;i++) { printf("%s\t%d\t%d\t%c\n",stud[i].name,stud[i].rollno,stud[i].marks,stud[i].grade); } for(i = 0;i<40;i++) printf("_");printf("\n"); getch();
return 0;
}
Output – Processing Student Marks and Display Results
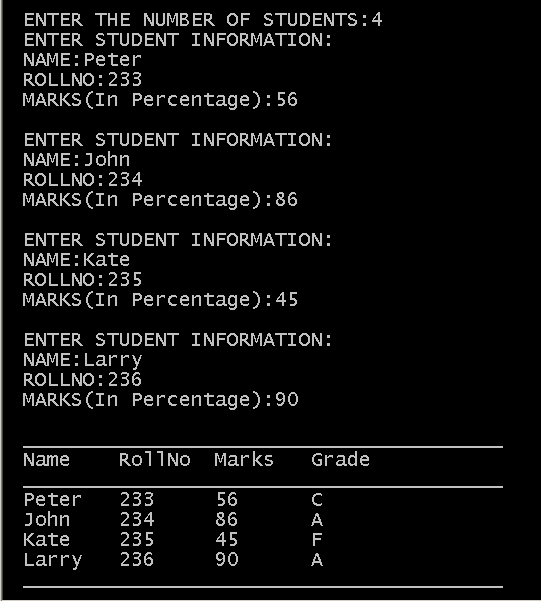
Comments
Post a Comment